Why Idempotency Can Save Your API (and Your Users)
The ability of a system to produce the same outcome, even if the same file, event or message is received more than once.
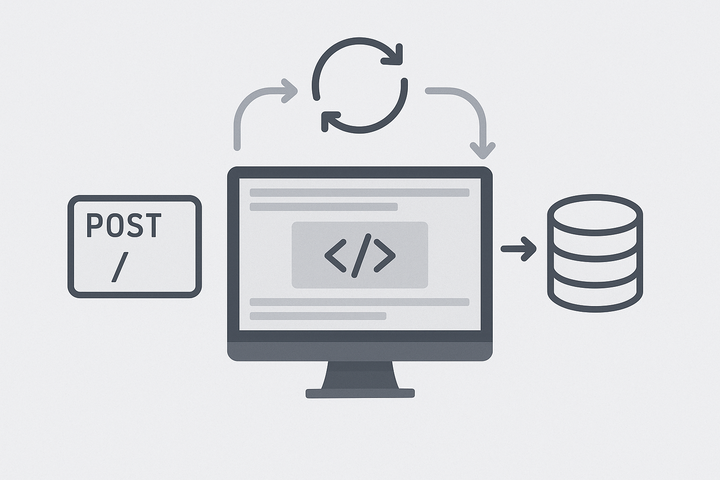
What is Idempotency?
In software development, idempotency refers to the ability of a system to produce the same result even if a particular operation is performed multiple times. This concept is especially critical in API design, where network instability, client retries, or unexpected errors can lead to duplicate requests being sent to a server.
Without proper handling, these repeated requests could cause unintended side effects—like charging a user multiple times or booking a reservation twice. Idempotency ensures that even if the same request is received more than once, the outcome remains consistent and predictable.
Let’s explore a practical example to see why this matters.
Example: Bank Transfer without Idempotency
Imagine a customer, Ana, initiates a bank transfer of 100 € to her friend Luis using a mobile banking app.
The app sends the following POST
request to the backend API:
{
"from_account": "ES1234567890",
"to_account": "ES0987654321",
"amount": 100
}
sequenceDiagram
participant Client as Ana (Mobile App)
participant Server as API Server
participant DB as Database
Client->>Server: POST /transfer {100€}
Server->>DB: Create transfer record (100€)
DB-->>Server: OK
Server-->>Client: Timeout / No response
Note over Client: Ana retries the request
Client->>Server: POST /transfer {100€}
Server->>DB: Create transfer record (100€)
DB-->>Server: OK
Server-->>Client: 200 OK
Note over DB: Luis receives 200€, Ana debited twice
Because Ana’s connection is unstable, she doesn’t get a response and the app either allows her to retry manually or automatically retries the same request. Since the server is not using any mechanism to detect duplicated requests, it processes both requests, resulting in two transfers of 100 €.
➡️ Outcome: Luis receives 200 €, and Ana is mistakenly debited twice.
This example highlights how critical idempotency is when dealing with financial operations. Without it, duplicate requests can lead to severe inconsistencies and a poor user experience.
As we’ve seen, things can go wrong very quickly. Now let’s see how idempotency comes to the rescue.
Idempotency to the rescue
One common solution is to use idempotency keys, a mechanism that allows APIs to recognize and safely handle duplicate requests. When a client sends a request, it includes a unique key (often a randomly generated string) in the request header. The server stores this key along with the response. If the client retries the same request with the same key, the server recognizes it and returns the original response instead of processing the request again. This mechanism prevents duplicate operations, such as double charges in payment systems.  
For example, a payment processing platform, implements idempotency keys to ensure that retrying a payment request doesn’t result in multiple charges. Clients include an Idempotency-Key in their POST requests. If a network failure occurs and the client resends the request with the same key, the server will return the original response, ensuring the customer is charged only once.  
{
"idempotency_key": "abc123", // ensures this request is processed only once
"from_account": "ES1234567890",
"to_account": "ES0987654321",
"amount": 100
}
sequenceDiagram
participant Client as Ana (Mobile App)
participant Server as API Server
participant DB as Database
Client->>Server: POST /transfer { amount: 100€, Idempotency-Key: abc123 }
Server->>DB: Check idempotency key
alt Not found
Server->>DB: Create transfer record (100€)
DB-->>Server: OK
Server->>DB: Store idempotency key
Server-->>Client: 200 OK
else Already exists
Server-->>Client: 200 OK (cached response)
end
Note over DB: Luis receives 100€, Ana debited once
When and Where to Use Idempotency
Now that we’ve seen how idempotency works in practice, let’s explore when you should actually use it—and when you can skip it.
Idempotency is not always required for every API endpoint, but it is crucial in scenarios where repeated requests could have harmful side effects. Here’s a quick guide:
✅ Use idempotency when:
- Processing payments or financial transactions
- Creating bookings or reservations
- Managing inventory or stock changes
- Sending transactional emails or messages
These operations often involve external systems or irreversible actions, so duplicate processing can be costly or confusing to users.
🚫 Not always necessary for:
- GET requests, which are already idempotent by design
- PUT and DELETE requests, which are also idempotent according to the HTTP spec (though you should still verify implementation)
- POST requests used for logging, analytics, or non-critical data submissions where retries don’t cause issues
Understanding where idempotency matters most helps you design more reliable APIs while keeping your system efficient and simple.
Summary Comparison
Scenario | Without Idempotency | With Idempotency |
---|---|---|
Payment Retry | Double charge | Single charge |
Booking Confirmation | Multiple reservations | Single reservation |
Inventory Adjustment | Overcount/Undercount | Accurate state |
In summary, incorporating idempotency keys into API design enhances reliability and predictability, safeguarding against unintended duplicate operations and improving the overall user experience.
In a world where reliability and user trust are key, idempotency is not just a best practice — it’s a necessity.